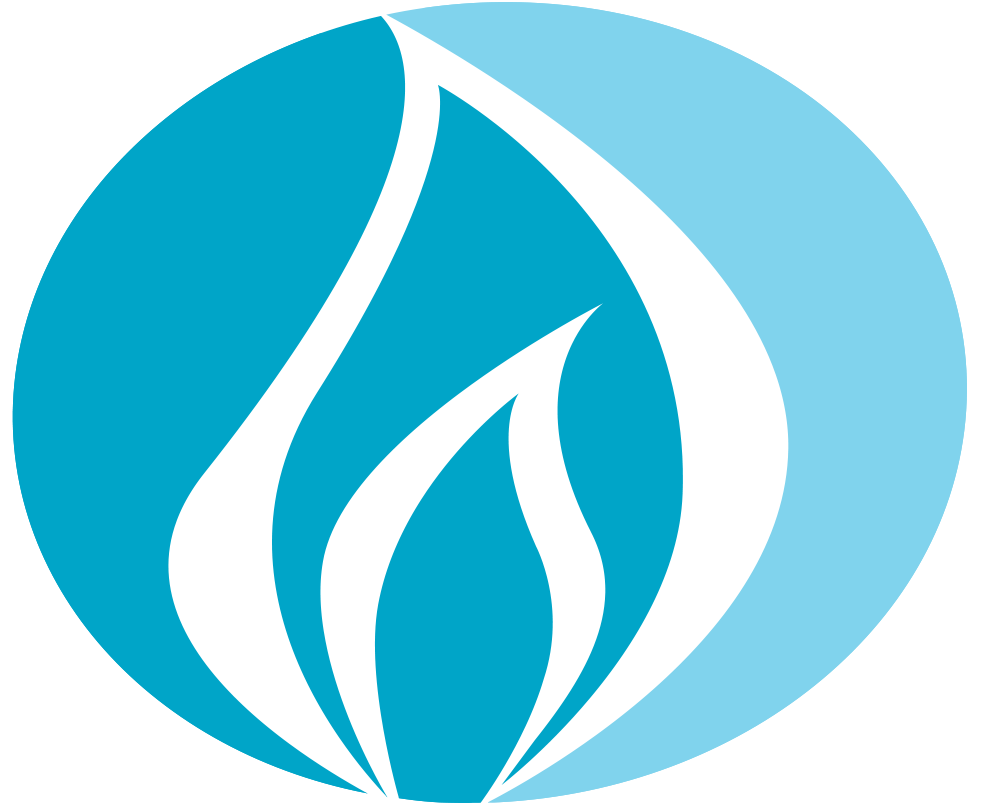
Convert all character vectors containing a set of values in a data.frame to factors.
Source:R/factorize.R
factorize_df.Rd
This function examines each column in a data.frame; when it finds a column composed solely of the values provided to the lvls
argument it updates them to be factor variables, with levels in the order provided.
This is an alternative to calling dplyr::mutate_at
with factor()
and identifying the specific variables you want to transform, if you have several repeated sets of responses.
Arguments
- dat
data.frame with some factor variables stored as characters.
- lvls
The factor levels in your variable(s), in order. If you have a question whose possible responses are a subset of another question's, don't use this function; manipulate the specific columns with
dplyr::mutate_at
.- ignore.case
Logical. If TRUE, will match without checking case, using the capitalization from the
lvls
parameter for the final output. If not provided, the function will provide a warning if it detects columns that would match without checking case but will NOT coerce them.
Examples
teacher_survey |>
factorize_df(lvls = c("Strongly Disagree", "Disagree", "Somewhat Disagree",
"Somewhat Agree", "Agree", "Strongly Agree"))
#> ℹ Changed the following columns to factors: `It's fair to expect students in
#> this class to master these standards by the end of the year.`, `One year is
#> enough time for students in this class to master these standards.`, `All
#> students in my class can master the grade-level standards by the end of the
#> year.`, and `The standards are appropriate for the students in this class.`
#> # A tibble: 20 × 5
#> timing It's fair to expect st…¹ One year is enough t…² All students in my c…³
#> <chr> <fct> <fct> <fct>
#> 1 Pre Disagree Disagree Agree
#> 2 Pre Disagree Somewhat Agree Agree
#> 3 Pre Somewhat Disagree Somewhat Disagree Strongly Agree
#> 4 Pre Disagree Strongly Disagree Strongly Agree
#> 5 Pre Agree Somewhat Disagree Strongly Disagree
#> 6 Pre Strongly Disagree Somewhat Agree Somewhat Disagree
#> 7 Pre Agree Strongly Agree Strongly Disagree
#> 8 Pre Agree Disagree Somewhat Agree
#> 9 Pre Strongly Disagree Disagree Somewhat Agree
#> 10 Pre Somewhat Agree Somewhat Disagree Agree
#> 11 Post Strongly Disagree Somewhat Agree Somewhat Disagree
#> 12 Post Somewhat Agree Disagree Somewhat Disagree
#> 13 Post Somewhat Disagree Somewhat Agree Strongly Disagree
#> 14 Post Strongly Agree Strongly Agree Disagree
#> 15 Post Strongly Agree Somewhat Disagree Strongly Disagree
#> 16 Post Disagree Disagree Agree
#> 17 Post Strongly Agree Agree Agree
#> 18 Post Strongly Agree Strongly Agree Disagree
#> 19 Post Somewhat Disagree Somewhat Agree Agree
#> 20 Post Somewhat Agree Strongly Agree Strongly Disagree
#> # ℹ abbreviated names:
#> # ¹`It's fair to expect students in this class to master these standards by the end of the year.`,
#> # ²`One year is enough time for students in this class to master these standards.`,
#> # ³`All students in my class can master the grade-level standards by the end of the year.`
#> # ℹ 1 more variable:
#> # `The standards are appropriate for the students in this class.` <fct>
# prints warning due to case mismatches:
teacher_survey |>
factorize_df(lvls = c("Strongly disagree", "Disagree", "Somewhat disagree",
"Somewhat agree", "Agree", "Strongly agree"))
#> Warning: ! Columns `It's fair to expect students in this class to master these standards
#> by the end of the year.`, `One year is enough time for students in this class
#> to master these standards.`, `All students in my class can master the
#> grade-level standards by the end of the year.`, and `The standards are
#> appropriate for the students in this class.` were NOT matched, but would
#> match if `ignore.case` was set to "TRUE"
#> Warning: ! No columns matched. Check spelling & capitalization of your levels.
#> # A tibble: 20 × 5
#> timing It's fair to expect st…¹ One year is enough t…² All students in my c…³
#> <chr> <chr> <chr> <chr>
#> 1 Pre Disagree Disagree Agree
#> 2 Pre Disagree Somewhat Agree Agree
#> 3 Pre Somewhat Disagree Somewhat Disagree Strongly Agree
#> 4 Pre Disagree Strongly Disagree Strongly Agree
#> 5 Pre Agree Somewhat Disagree Strongly Disagree
#> 6 Pre Strongly Disagree Somewhat Agree Somewhat Disagree
#> 7 Pre Agree Strongly Agree Strongly Disagree
#> 8 Pre Agree Disagree Somewhat Agree
#> 9 Pre Strongly Disagree Disagree Somewhat Agree
#> 10 Pre Somewhat Agree Somewhat Disagree Agree
#> 11 Post Strongly Disagree Somewhat Agree Somewhat Disagree
#> 12 Post Somewhat Agree Disagree Somewhat Disagree
#> 13 Post Somewhat Disagree Somewhat Agree Strongly Disagree
#> 14 Post Strongly Agree Strongly Agree Disagree
#> 15 Post Strongly Agree Somewhat Disagree Strongly Disagree
#> 16 Post Disagree Disagree Agree
#> 17 Post Strongly Agree Agree Agree
#> 18 Post Strongly Agree Strongly Agree Disagree
#> 19 Post Somewhat Disagree Somewhat Agree Agree
#> 20 Post Somewhat Agree Strongly Agree Strongly Disagree
#> # ℹ abbreviated names:
#> # ¹`It's fair to expect students in this class to master these standards by the end of the year.`,
#> # ²`One year is enough time for students in this class to master these standards.`,
#> # ³`All students in my class can master the grade-level standards by the end of the year.`
#> # ℹ 1 more variable:
#> # `The standards are appropriate for the students in this class.` <chr>